Example
1. Entry, Pixmap & Dialog Widgets
By Ishan Chattopadhyaya
Introduction
This example of the building of
a program using wGLADE will show you in easy steps how to:
- Use wGLADE and create an interface
- Build the source code using wGLADE
- Edit the source code manually (notepad or any other plain text editor)
In this tutorial, you will see how to create an
interface and how to link some common widgets with one another.
Creating the interface
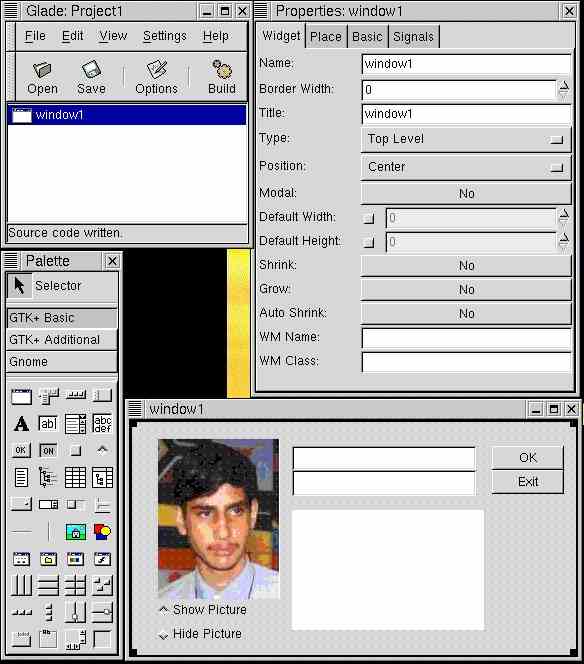
The GLADE Environment
(Click for a larger view)
|
Open wGLADE. You will see a number
of component windows of wGLADE. They are wGLADE (Main), Palette, Properties,
etc. (See figure above for an example environment) Proceed as
follows:
- Click on Window icon in the Palette
(the first icon). By this, a window is created which will form the main window
for this tutorial program. You’ll see that window1 is added to the
wGLADE window. Double click it. This displays the windows for
editing.
- Select Fixed Position in the
Palette (you’ll find it in the rightmost column and the eighth row
down) and click in the window1.
[Note for other programmers: Using a fixed position
container is easier for beginners rather than using vertical and horizontal
boxes etc. Besides, all who have previous experience with Microsoft
Visual Basic/C++ or Power Builder will find it more comfortable
to adjust. Using vertical and horizontal box containers can be left
for a later stage in the course for a beginner learning wGLADE.]
- Add two text entry
widgets, two buttons, one pixmap and two radio
buttons to your container in the window1. Also add a list
widget.
[Tip: You can move your mouse over the icons in the
palette and wait for a second for the ‘tool tips’ to popup. Take a while
exploring these tool tips so you are comfortable with the development
environment.]
- Name one button as
BT_OK and label it as OK. Name the other button as
BT_EXIT and label it as Exit. (You can select the
button and change the Name and Label from the Properties
window.) Similarly name the two text entry boxes as entry1 and
entry2 . Name the radio buttons as radiobutton1
and radiobutton2 and label them as Show Picture
and Hide Picture respectively. Name the list as list1
. Name the pixmap as pixmap1 and assign any xpm file
of your choice to it.
- Click on the window1
on the wGLADE window and from the Properties window, select
suitable width and height for your window.
- Your interface should
now look somewhat like this:
- Now select your
OK button. Click on the Signals tab on the Properties
window. Here, add a signal as clicked
(Click at "..." button and select "Clicked" in the windows that
pops up and then click Add in the Properties window. See the figures
below! ) with the suggested function name on_BT_OK_clicked
. Do the same for the other button. For the radio
buttons, add the released signals. In both the occasions,
accept the default function name which wGLADE provides.
Click at window1 from the wGLADE window to change to properties
of the Window. In the signals tab of the property window, add a
destroy signal.
Adding the Signals (After
Clicking "..." button)
|
Adding the Signals (After
Clicking Add button)
|
- Now save your project. The Project Options dialog will appear. Here
select the path of your project, e.g. C:\Projects\First . Select C
as the programming language. Once you are through with Project Options, click
at the Build Source button from the toolbar for wGLADE to write the code for
your interface.
Editing the code
Now that we have successfully created the interface and built
the source for it, the time has come to edit the code. Since wGLADE is not an
Integrated Development Environment (IDE) like Visual Basic, Visual C++ etc.,
you will need to edit the code using an external text editor. Many programmers
use notepad as their text editor for coding, but I prefer using a specialist
programmer's editor which has a multiple document interface (MDI), e.g. Crimson
Editor from http://geocities.com/crimsonware.
The choice of editor is up to you!
Whichever text editor it might be, fire it up. Assuming that
during building your source you gave C:\Projects\First
as the directory of your project, open the file C:\Projects\First\src\callbacks.c
in your editor. This is the file where all the callback functions are written.
What I mean is that you can assign any functions to the widgets that you connected
during adding the signals. So, this file is mainly responsible for the interactivity
between the widgets, e.g. buttons, etc. Begin by inserting #include
<string.h> at the top of the file.
Scroll down the file until you come across the function on_BT_EXIT_clicked.
In the space that is provided within the function, add a function: gtk_main_quit();
Your function should now look like this:
void |
on_BT_EXIT_clicked
(GtkButton *button, |
gpointer user_data) |
{
gtk_main_quit();
}
|
[Note: The gtk_main_quit()
function causes the program to end. Another alternate function
exit(0); can also be used for the same purpose. As you wil have
seen in the previous example, the gtk_main_quit function can be included
automatically from within wGLADE. This method simply represents an alternative.]
Do the same for the on_window1_destroy function.
That is add gtk_main_quit() function there also. This ensures
that when the window is closed using the 'X' button on the upper
right, the program ends.
Similarly, add the following code to the on_BT_OK_clicked
function:
void |
on_BT_OK_clicked
(GtkButton *button, |
gpointer user_data) |
{
/* INITIALIZATIONS
START HERE */
GtkEntry *two; |
// this will be used for the second entry |
GtkWidget * listitem; |
// These will |
gchar str[50]; |
// be used |
gchar * p_str
= str; |
// for the list |
GtkWidget *dialog,
*label, *okay_button; |
// These will be used
for the dialog |
char *text; |
|
/* COPYING THE TEXT ENTERED IN ENTRY1 TO THE LIST WIDGET */
GtkWidget
* entry = lookup_widget(GTK_WIDGET(button), "entry1"); |
// Getting
pointers to |
GtkWidget
* list = lookup_widget(GTK_WIDGET(button), "list1"); |
//
entry1 & list1 |
|
|
strncpy(p_str,
gtk_entry_get_text(GTK_ENTRY(entry)),50); |
// Storing
the text of entry1 in the variable p_str |
|
|
listitem
= gtk_list_item_new_with_label (p_str); |
// Adding p_str to the list |
gtk_container_add
(GTK_CONTAINER (list), listitem); |
|
|
|
gtk_widget_show(listitem); |
// Showing the updated list |
/* COPYING THE TEXT
ENTERED IN entry1 TO entry2 */
text = gtk_entry_get_text
(GTK_ENTRY (entry)); |
// obtain the text from the widget |
two = GTK_ENTRY(lookup_widget(
GTK_WIDGET (button),
"entry2")); |
// obtain the pointer to the second GtkEntry widget |
gtk_entry_set_text(two,
text); |
// set the text for the widget |
/* SHOWING
A DIALOG BOX WITH THE TEXT OF entry1 IN IT */
dialog
= gtk_dialog_new(); |
|
label
= gtk_label_new (text); |
// Create the widgets of the dialog box |
okay_button
= gtk_button_new_with_label("Okay"); |
|
|
|
gtk_signal_connect_object(GTK_OBJECT (okay_button),
"clicked", gtk_widget_destroy, GTK_OBJECT(dialog));
|
// Ensure that the dialog box is destroyed when the user
clicks ok. |
gtk_container_add
(GTK_CONTAINER (GTK_DIALOG(dialog)->action_area), okay_button); |
// Add the Okay Button to the dialof |
|
|
gtk_container_add
(GTK_CONTAINER (GTK_DIALOG(dialog)->vbox), label); |
// Add the label |
gtk_widget_show_all
(dialog); |
// Show everything the dialog. |
}
|
Now add the following
code to the two radiobutton functions, like this:
void |
on_radiobutton1_released
(GtkButton *button, |
gpointer user_data) |
{
GtkPixmap
*pix; |
// Initialisation |
pix
= GTK_PIXMAP(lookup_widget(GTK_WIDGET (
button), "pixmap1")); |
// Getting
the pointer to the pixmap |
gtk_widget_show(
GTK_WIDGET (pix)); |
// Show
the pixmap |
}
void |
on_radiobutton2_released
(GtkButton *button, |
gpointer user_data) |
{
GtkPixmap
*pix; |
// Initialisation |
pix
= GTK_PIXMAP(lookup_widget(GTK_WIDGET (
button), "pixmap1")); |
// Getting
the pointer to the pixmap |
gtk_widget_hide(
GTK_WIDGET (pix)); |
// Hide
the pixmap |
}
|
This piece of code is
quite simple to understand. What the on_BT_OK_clicked function does is
as follows:
- Copies the text entered
by the user in entry1 to entry2
- Copying the same
text to the list widget as an item
- Creating a dialog
box displaying the text of the entry1
Now that the coding part is done,
now you have to proceed compiling your project. This is carried out in exactly
the same way as mentioned in the Getting Started
page.
All the examples in these exercises
are available in one file (Projects.zip)
which you can download.
Overview of this tutorial
Simply follow the same steps that
you used to compile your first project (Getting Started). This
method is the one to be used for every project that you will build with wGLADE.
>> Example 2: Text Handling >>
Download Zipfile of all the examples
(63 KB)
